New interoperability for Nintendo Amiibo NFC toys
Demonstration: writing on a Raspberry Pi, reading on Windows
This demo shows new interoperability of Nintendo Amiibo NFC toys. Previously, only Nintendo hardware and games had the ability to write data to Amiibo NFC toys.
Any NFC reader can read the ATQA, SAK and UID of an NFC toy. These let you tell it's an Amiibo, and its unique ID.
Any NFC reader can also read the data from an Amiibo.
By knowing the algorithm used to set the write password (PWD), we can interoperably write our own data to an Amiibo NFC toy.
This video first shows a program which computes the PWD and uses it to write new, custom text to an Amiibo NFC toy. The program is running on a standard Raspberry Pi computer, using a commercial, off-the-shelf, NFC add-on (the ITEAD PN532 NFC module).
Then, a standard Windows 10 laptop is shown, using a commercial, off-the-shelf, USB NFC reader (the Identiv SCL3711), reading the custom text from the toy, using the open source Google Chrome App NFC Library.
The video has artificial delays inserted so the on-screen explanations can be read; computing the PWD and reading and writing to the NFC toy are nearly instantaneous otherwise.
Read Writing your own data to a Nintendo Amiibo NFC toy to see a step-by-step workflow similar to the demo video, using standard software available on any Mac or Linux computer.
Computing the PWD
In 2015, researcher Marcos Del Sol Vives figured out how to derive the PWD from the UID. It's since been informally published across the internet, and the bitwise XOR math involved is simple enough to do by hand. Knowing the PWD allows any compatible NFC device to write to the toy.
A clean room description of the algorithm is as follows:
The Amiibo UID is seven digits in hexadecimal, e.g.
04:52:D7:52:01:49:81
. We'll refer to them asUID 0
throughUID 6
. The resulting PWD is four digits in hexadecimal, e.g.aa:83:b1:d5
. We'll refer to them asPWD 0
throughPWD 3
.
UID 1
xorUID 3
xoraa
=PWD 0
UID 2
xorUID 4
xor55
=PWD 1
UID 3
xorUID 5
xoraa
=PWD 2
UID 4
xorUID 6
xor55
=PWD 3
e.g.
52
xor52
xoraa
=aa
d7
xor01
xor55
=83
52
xor49
xoraa
=b1
01
xor81
xor55
=d5
If you have the UID for an Amiibo NFC toy, this printable PDF worksheet (8.5"x11", color) walks you through the math to figure out the PWD for that toy by hand.
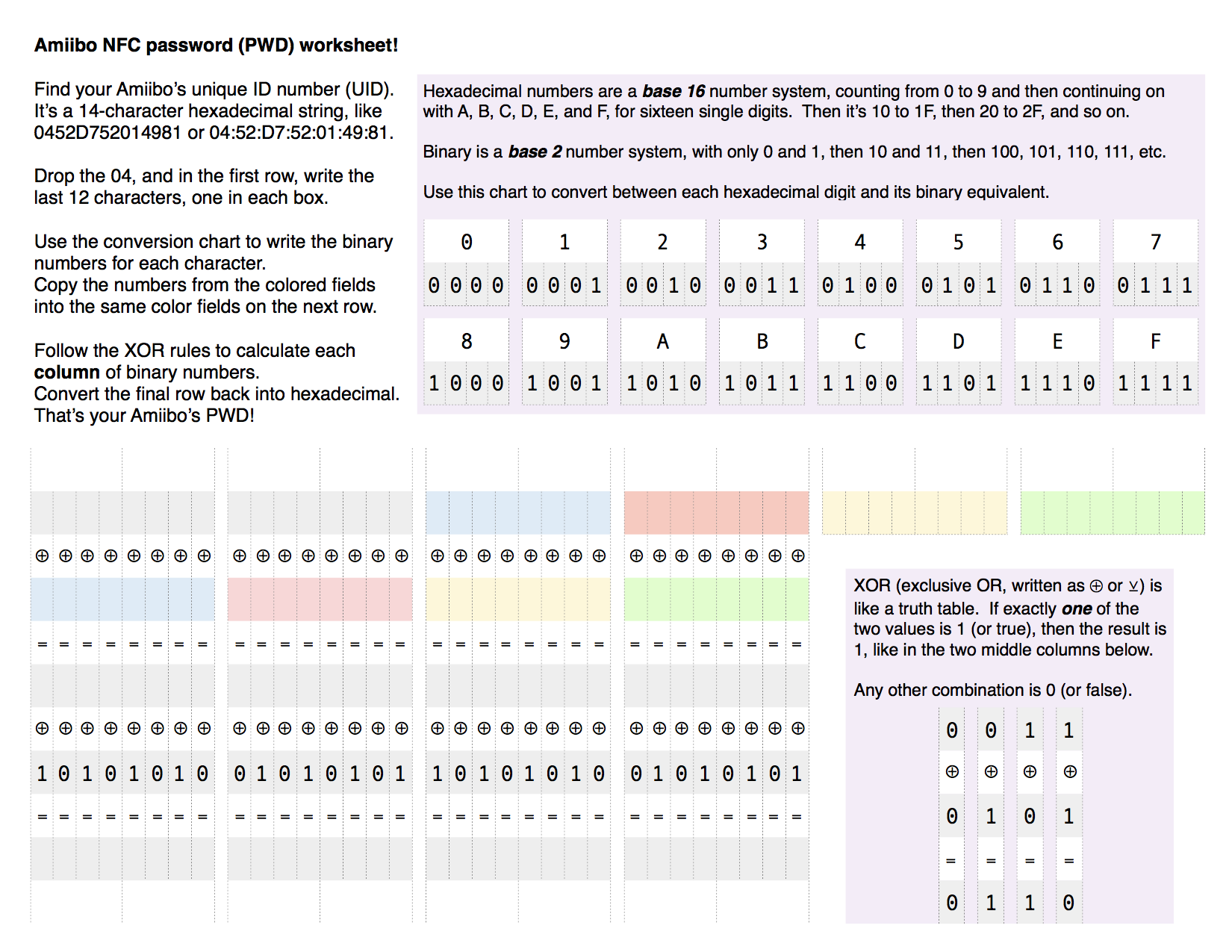
The first page works through the same example shown above and in the video. The second page is a blank worksheet for you to use.
An example of this implemented in Python 2 is as follows:
#!/usr/bin/python
## pwd215.py - Compute a PWD
##
## Written in 2016 by Vitorio Miliano
##
## To the extent possible under law, the author has dedicated all
## copyright and related and neighboring rights to this software to
## the public domain worldwide. This software is distributed without
## any warranty.
##
## You should have received a copy of the CC0 Public Domain
## Dedication along with this software. If not, see
## <http://creativecommons.org/publicdomain/zero/1.0/>.
import os, re, sys
uidre = re.compile('^04[0-9a-f]{12}$', re.IGNORECASE)
def calc_keya(uid, sector):
if uidre.match(uid) is None:
raise ValueError('invalid UID (seven hex bytes)')
if sector < 0 or sector > 134:
raise ValueError('invalid sector (0-134)')
intvals = [int(uid[i] + uid[i+1], 16) for i in range(0, len(uid), 2)]
intkey = [intvals[1] ^ intvals[3] ^ 170, intvals[2] ^ intvals[4] ^ 85, intvals[3] ^ intvals[5] ^ 170, intvals[4] ^ intvals[6] ^ 85]
key = ''.join(format(i, '02x') for i in intkey)
return key
if __name__ == '__main__':
if len(sys.argv) > 1:
print calc_keya(sys.argv[1], 0)
Use at your own risk
I don't personally know what happens if you change or replace the data on an Amiibo and then try to use it with a Nintendo game. At the time I wrote this code, I did not own a Nintendo console with NFC support; I just liked collecting the figures. However, other researchers have indicated the figures continue to function (as new figures).